hi does anybody know where i can find jdk 8 as im unable to fix an error which is due to me having a high jdk :/
↧
Jdk 8
↧
RS3 [NXT] Disabling ISAAC + Reverse-engineering tutorial
Why am I doing this... How to disable ISAAC in the NXT client
Or: How to get started with Ghidra, finding your way, patching instructions, exporting the binary. You don't have to actually disable ISAAC, you can follow along. Or you are redirected from future tutorials as a reference as to how to work with Ghidra.
This is a long tutorial
As with my NXT to login screen tutorial, this can get technical. However, I am aiming to keep it as simple as I can so that everyone can follow along and learn about Ghidra and NXT.
In this tutorial, the following will be handled, in order:
- How to open your binary with Ghidra
- How to find the jag::Isaac::Init method in the client using memory searching
- How to find the jag::Isaac::Generate method
- How to create a structure for our jag::Isaac struct
- How to refactor our jag::Isaac::Generate method
- How to patch jag::Isaac::Generate to disable ISAAC
- How to export our binary
This tutorial will NOT cover the following:
- How to generate the hash for the patched binary
- How to compress the patched binary
These are things that are already in my patcher. I do expect you to be able to understand the patcher before making a server with NXT. The above two are literally functions in my patcher. If you can't figure that out, this tutorial is not for you, and you should focus on learning more about programming. NXT is complex, and this tutorial does not cover packet identification, which is a way more complex process.
A heads-up
So, first of all, I am not sure how smart doing this is. As long as you don't disable RSA your passwords aren't stored plaintext, but it's still not ideal to do this. Unfortunately though, many frameworks don't support ISAAC, and sometimes it's even a huge pain in the butt to add support for ISAAC (Looking at you, Matrix). I highly recommend avoiding this last-resort, as it's technical, difficult, and I am not sure yet if this will break things.
Basically, what we're going to do is patch some instructions in the jag::Isaac::Generate method. We're going to have to use some assembly tricks in order to preserve instructions, as we don't have much space (The client I tested it on only had 4 bytes at the end of the function).
Finally, for future reverse engineering, sometimes the compiler inlines functions. This means instead of making a function, the instructions of the functions are added to every place the function would have been called. This also makes reverse engineering harder.
Prerequisites
We're going to need a NXT client and Ghidra. You can get Ghidra at https://ghidra-sre.org/
Project setup
Once you have downloaded Ghidra, open it up and make a new project. Give it any name you want. Then, drag the NXT client into the window, importing it. Just click OK if you face any dialogue, the default settings should be good. Please don't forget to extract the client from the LZMA archive. The client should be 7-8MB. If it's around 2MB, extract it, and use the extracted client.
Your Ghidra window should look something like this, by this point:
![]()
Analyizing the binary
Now, double-click your binary. In my case, rs2client_raw.exe. This will open up the editor. The first time you open up a binary, you will be faced with a popup box asking if you want to analyze the binary. Once analysis is done, you won't have to analyze the binary anymore the next time you open it. Click yes, and then click Analyze. This will take a while (Up to 40-45 minutes depending on your computer). Click it now and continue reading so you know what's up.
![]()
Allow Ghidra to take its time. Binaries are incredibly complex, unlike Java, the only data remaining in binaries are raw instructions. No class structures, no function start positions, no function end positions, literally only raw instructions. This is what makes reverse engineering so difficult too. If you're going to get serious about NXT, this tutorial will be a good place to start off, as it introduces you into how to get started with Ghidra.
Once Ghidra is completely done analyzing the binary, you'll get a warning about Ghidra being unable to locate the PDB file. It's impossible to get this file though, unless Jagex leaks it. Click OK, and we can continue with the tutorial.
Searching for jag::Isaac::Init using memory searching
As mentioned before, binaries are incredibly difficult to look through. While Ghidra has a decompiler, you can't really look through the binary by looking for code. This restricts our options on how we can find methods. That's also why we don't directly find jag::Isaac::Generate, but find it through jag::Isaac::Init. The same goes for many other functions - you'll have to be smart about it. This is not Java, this is far more complicated.
Anyway, there's one constant in the jag::Isaac::Init method we can use to find the method, which is used in several methods. We'll have to figure out which function is correct, and which is not. The constant we're looking for has hex value 0x9e3779b9. In Ghidra, press S on your keyboard to open the Search Memory popup. Alternatively, click Search in the top bar, and click Memory. In Search Value enter 9e3779b9. Select Hex format, Loaded Blocks and Search All. Finally, click search:
![]()
We're only interested in MOV operations, so we can sort by Code Unit in the results dialog. This will allow us to search more efficiently. We're looking for a function with several while loops, no nested loops, and a lot of bitshifting. At the end of the function there should be another function call. There's should also be no if statements. The end of the function in my binary looked like this:
![]()
Cool, now that we found the jag::Isaac::Init method, we can name it! Scroll all the way up in the decompiler and select the function name, now press L on your keyboard and enter jag::Isaac::Init
![]()
Now, Init is the function name, in the namespace jag::Isaac. We're naming things similarly as to how we would name things in Java, following a certain hierarchy going from a wide scope to a small scope (jag is a big scope, containing many things, Isaac is a way smaller scope and contains only Isaac related things)
Finding the jag::Isaac::Generate method
Nothing much to find, honestly. Remember how there must be a function call at the bottom of our jag::Isaac::Init function? That is our jag::Isaac::Generate method!
![]()
Double-click it to go to the function.
![]()
NOTE: If the function does not look like that, please PM me. It could be I made a mistake and that there's two similar functions that do different things. In which case I need to know so I can update this tutorial
First things first, let's rename the function so that we know which function we're dealing with again. Select the function name in the decompiler, press L and enter jag::Isaac::Generate. Now we can get back to this method easily through the Symbol Tree
![]()
Creating a struct for our jag::Isaac struct
We're decompiling the NXT binary as if it were C, so instead of using classes, we're using structs (To keep things simple). Ghidra does have support to create class structures, but I won't cover that in this tutorial.
In our jag::Isaac::Generate function, scroll to the top of the function and rename param_1 to this. Select param_1 and click L on your keyboard to rename a variable.
Now, this field probably has a random data type, even though we know it's a struct. So let's create a struct for this data type. Right-click either the parameter type or the parameter name and select Auto Create Structure. Ghidra will now automatically create a struct that most of the time has the right size and some of the fields already identified.
![]()
Our struct is now called astruct, but we want it to have an identifiable name. Right-click astruct in the decompiler and click Edit Data Type. This will open a popup that looks something like this:
![]()
At the bottom there's some fields, we're mainly interested in the Name field. Re-name the struct to jag::Isaac. The size, most of the time, is correct. Now, close the type editor and you'll see our function's prototype is now very readable:
![]()
Refactoring our method and struct
Our decompiler window now looks slightly different, with a lot of arrows and fields instead of array indices/pointer magic going on:
![]()
Now comes the fun part - refactoring our Isaac struct. We're now going to be cross-referencing with a normal Isaac implementation:
(Source: https://rosettacode.org/wiki/The_ISAAC_Cipher#Java)
As you can see, in my decompiler window, line 12 is incrementing something by one, and is used in line 14 to increment another field. So we already know which field is bb and cc. We can rename these fields through the Data Type Editor, or just through the decompiler. Select the variable names, and press L. Now enter the proper names:
![]()
On line 36 we can see a bitwise XOR operation, which looks a lot like the aa = aa ^ (aa *something*) going on in the switch statement above, so we can rename that field to aa.
![]()
Based on that, we can also figure out which field is mm - in my code that's field_0x404, as on line 39 aa is incremented using mm. So let's rename that to mm.
![]()
Now, that looks quite ugly. Let's open our data editor and see what's going on. We know mm is an array that holds 256 ints. Go to the top of the function, right-click jag::Isaac (The parameter type), click Edit Data Type and in Search enter mm, then press enter:
![]()
As you can see, Ghidra identified field mm to be an uint, but not a uint array! Let's change it. Let's double-click the DataType column for mm and enter uint[256]:
![]()
It won't let us press enter. At the bottom, an error pops up uint[256] doesn't fit within 4 bytes, need 1024 bytes. The field below is identified as uint too, causing this error (In my editor at offset 1032). So let's un-identify that field. Press ESC to cancel setting the data type of mm. Now click the field below (For me, offset 1032). Then press C on your keyboard to Clear the field. This sets the bytes to undefined:
![]()
Now, let's try to set the type of mm to an uint[256]:
![]()
That seems to have done the trick!
![]()
Now, there's still some unresolved fields we have to resolve. Let's get on with that! Around line 44 in my decompiler there's this code:
![]()
Interestingly enough, the variable puVar6 is set to this->mm at the beginning, which is an array. Also, interestingly enough, hex 0x100 is decimal 256, and since it's an array of uints (size = 4 bytes), it's setting data 1024 bytes before puVar6. It also increments the memory address of puVar6 each time by 4 bytes (Once again, +1 = 4 bytes as the data type of the array is 4 bytes). Based on this we can conclude there's another array of 256 values right before this array. The only unidentified array that remains is the results array. So let's open our data editor again and scroll up all the way:
![]()
And as you can see, there's already an uint that Ghidra found. Now, let's change the type of this field to an uint[256] just like we did to mm. I have also renamed the field to results by double-clicking the name column:
![]()
We're almost done completely refactoring the jag::Isaac struct. Only one field remains, which is our remaining field, indicating how many numbers remain before new data must be generated. There's also only enough for one more int, so let's give the field at offset 0 data type uint and let's name it remaining. Now our struct is fully refactored.
![]()
NOTE: Not everything is an int or uint, sometimes fields are char or short. You don't know for sure, but I knew for sure that remaining is of type int. Also the signing on the above fields may be wrong, I just went with what Ghidra picked. The most important thing is having the field names.
Wew. That was definitely a long section, the refactoring part.
Patching ISAAC out
Alright, so now it's time to actually disable ISAAC. For this, we'll be delving into assembly. Remember how the statement here sets a value in the results array?
![]()
If we set that to 0, that means every time we get a value for the ISAAC, we get a 0, which means every opcode is offset by 0, effectively removing ISAAC from the protocol, allowing us to be super lazy and making supporting frameworks like Matrix a lot easier.
The question is how we do it, though. Let's click the code in the decompiler, and it'll jump to the related assembly code.
![]()
The instruction we're interested in is MOV dword ptr [R9 + -0x400], EDX. This sets the value of dword ptr [R9 + -0x400] to the value of EDX. EDX and R9 are registers (You can google them - assembly x64 registers). Sadly, if we patch this to MOV dword ptr [R9 + -0x400], 0x0, we'll use too many bytes, so that approach is impossible.
We can try to work out if we can change the value of EDX though. It gets assigned and then added to in the first two instructions of the above screenshot. So while we can't do puVar6[-0x100] = 0x0 directly, we can cheat our way there. Instead of doing ADD EDX,R10D we can do XOR EDX,EDX. This is a very memory-efficient way of setting a field to 0, requiring only 2 bytes!
Right-click the ADD EDX,R10D code and click Patch Instruction. You will get a popup about assembler rating, just click OK. It will then construct the assembler. After a bit we can type:
![]()
Since we want to set the value in the register EDX to 0, we do XOR in the operator, and as operands, we enter EDX,EDX
Note: Operator = the field on the left. Operand = the field on the right
![]()
Now press enter to patch the instruction. But now we have a problem. The previous MOV instruction required 3 bytes, but the XOR only requires 2 bytes. So we have a leftover byte! Thankfully, we can just patch it to NOP, meaning it'll just get skipped.
![]()
Right-click the stray byte, Path Instruction and enter NOP in the operator. Leave the operand field empty. Finally, press enter to patch the instruction.
![]()
Finally, as you can see in the decompiler window, we see some changes:
![]()
The results array is now completely set to zeroes! It doesn't really matter that we broke the mm field - we're breaking ISAAC anyway. Now, everything is set to zero and packet opcodes will be offset by 0 all the time, both for incoming and outgoing packets.
Note: You'll still have to decrypt the XTEA block during login which follows the RSA block, it contains the username. We only patched out the ISAAC stuff, not the XTEA stuff during login ;)
Exporting the binary
First, save the project. Press CTRL+S or the save button in the top-left of the screen. Then click File (Top left of screen)->Export Program Or press O on your keyboard.
Now, set the format to Binary. Then, select an output file. Ghidra will automatically append .bin to the filename, but you can rename it to .exe. Then, click OK and you're good to go!
![]()
You can now re-calculate your CRC and re-generate your hash, compress the client, and test it out. If you crash due to some stack error, you likely messed something small up. Cry it out and start over. I've done that plenty times.
Finally
I am interested in making more tutorials on NXT, so if you have any ideas, please drop them below. Also, I've only tested this tutorial on the 910 client, so things may look slightly different in other clients. If you're really stuck on another version, please let me know and send me your binary. I can take a look for you. I'm interested in helping the scene out as a whole, and it seems like frameworks have a hard time supporting ISAAC, so this is my first push in the back for those servers. ISAAC can cause a lot of weird issues, especially with the NXT client.
This tutorial also attempts to cover some of the useful parts of reverse engineering, so even if you don't want to patch ISAAC out, it contains a lot of background information that will be required in future tutorials.
Or: How to get started with Ghidra, finding your way, patching instructions, exporting the binary. You don't have to actually disable ISAAC, you can follow along. Or you are redirected from future tutorials as a reference as to how to work with Ghidra.
This is a long tutorial
As with my NXT to login screen tutorial, this can get technical. However, I am aiming to keep it as simple as I can so that everyone can follow along and learn about Ghidra and NXT.
In this tutorial, the following will be handled, in order:
- How to open your binary with Ghidra
- How to find the jag::Isaac::Init method in the client using memory searching
- How to find the jag::Isaac::Generate method
- How to create a structure for our jag::Isaac struct
- How to refactor our jag::Isaac::Generate method
- How to patch jag::Isaac::Generate to disable ISAAC
- How to export our binary
This tutorial will NOT cover the following:
- How to generate the hash for the patched binary
- How to compress the patched binary
These are things that are already in my patcher. I do expect you to be able to understand the patcher before making a server with NXT. The above two are literally functions in my patcher. If you can't figure that out, this tutorial is not for you, and you should focus on learning more about programming. NXT is complex, and this tutorial does not cover packet identification, which is a way more complex process.
A heads-up
So, first of all, I am not sure how smart doing this is. As long as you don't disable RSA your passwords aren't stored plaintext, but it's still not ideal to do this. Unfortunately though, many frameworks don't support ISAAC, and sometimes it's even a huge pain in the butt to add support for ISAAC (Looking at you, Matrix). I highly recommend avoiding this last-resort, as it's technical, difficult, and I am not sure yet if this will break things.
Basically, what we're going to do is patch some instructions in the jag::Isaac::Generate method. We're going to have to use some assembly tricks in order to preserve instructions, as we don't have much space (The client I tested it on only had 4 bytes at the end of the function).
Finally, for future reverse engineering, sometimes the compiler inlines functions. This means instead of making a function, the instructions of the functions are added to every place the function would have been called. This also makes reverse engineering harder.
Prerequisites
We're going to need a NXT client and Ghidra. You can get Ghidra at https://ghidra-sre.org/
Project setup
Once you have downloaded Ghidra, open it up and make a new project. Give it any name you want. Then, drag the NXT client into the window, importing it. Just click OK if you face any dialogue, the default settings should be good. Please don't forget to extract the client from the LZMA archive. The client should be 7-8MB. If it's around 2MB, extract it, and use the extracted client.
Your Ghidra window should look something like this, by this point:
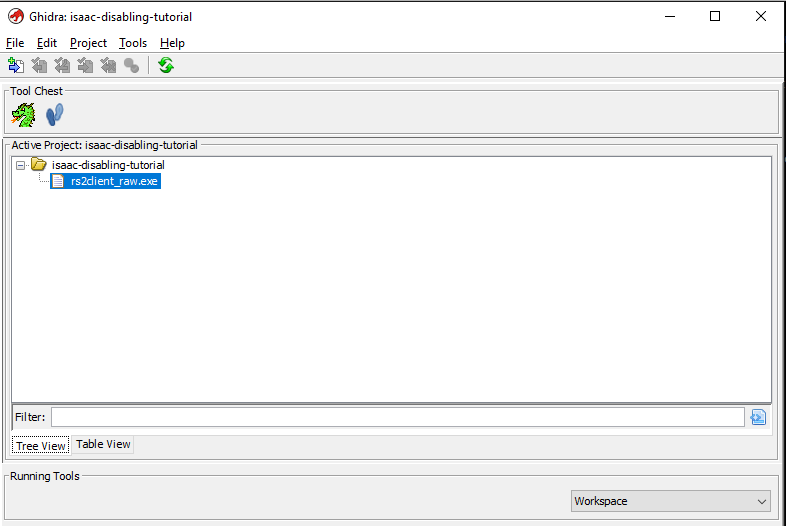
Analyizing the binary
Now, double-click your binary. In my case, rs2client_raw.exe. This will open up the editor. The first time you open up a binary, you will be faced with a popup box asking if you want to analyze the binary. Once analysis is done, you won't have to analyze the binary anymore the next time you open it. Click yes, and then click Analyze. This will take a while (Up to 40-45 minutes depending on your computer). Click it now and continue reading so you know what's up.
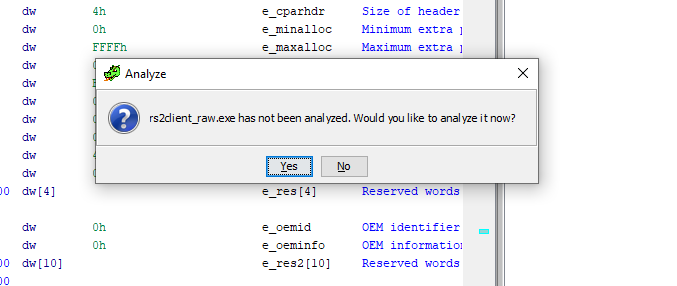
Allow Ghidra to take its time. Binaries are incredibly complex, unlike Java, the only data remaining in binaries are raw instructions. No class structures, no function start positions, no function end positions, literally only raw instructions. This is what makes reverse engineering so difficult too. If you're going to get serious about NXT, this tutorial will be a good place to start off, as it introduces you into how to get started with Ghidra.
Once Ghidra is completely done analyzing the binary, you'll get a warning about Ghidra being unable to locate the PDB file. It's impossible to get this file though, unless Jagex leaks it. Click OK, and we can continue with the tutorial.
Searching for jag::Isaac::Init using memory searching
As mentioned before, binaries are incredibly difficult to look through. While Ghidra has a decompiler, you can't really look through the binary by looking for code. This restricts our options on how we can find methods. That's also why we don't directly find jag::Isaac::Generate, but find it through jag::Isaac::Init. The same goes for many other functions - you'll have to be smart about it. This is not Java, this is far more complicated.
Anyway, there's one constant in the jag::Isaac::Init method we can use to find the method, which is used in several methods. We'll have to figure out which function is correct, and which is not. The constant we're looking for has hex value 0x9e3779b9. In Ghidra, press S on your keyboard to open the Search Memory popup. Alternatively, click Search in the top bar, and click Memory. In Search Value enter 9e3779b9. Select Hex format, Loaded Blocks and Search All. Finally, click search:
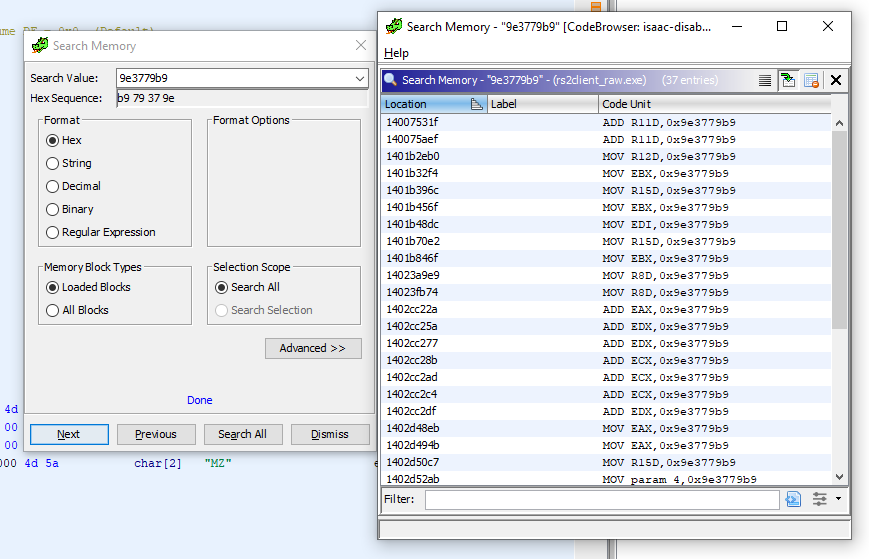
We're only interested in MOV operations, so we can sort by Code Unit in the results dialog. This will allow us to search more efficiently. We're looking for a function with several while loops, no nested loops, and a lot of bitshifting. At the end of the function there should be another function call. There's should also be no if statements. The end of the function in my binary looked like this:
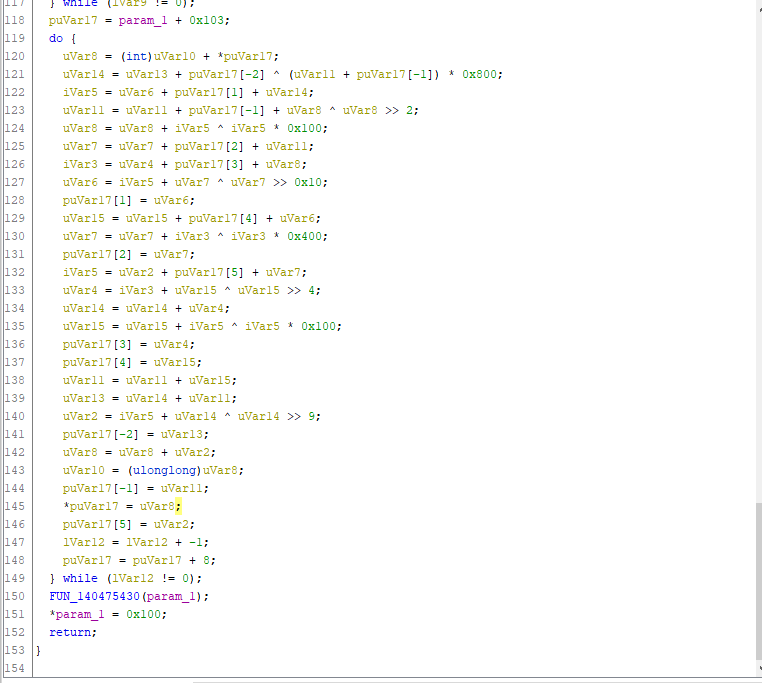
Cool, now that we found the jag::Isaac::Init method, we can name it! Scroll all the way up in the decompiler and select the function name, now press L on your keyboard and enter jag::Isaac::Init
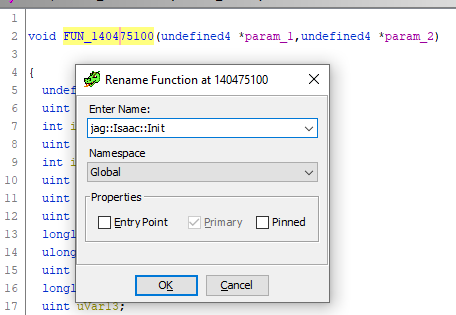
Now, Init is the function name, in the namespace jag::Isaac. We're naming things similarly as to how we would name things in Java, following a certain hierarchy going from a wide scope to a small scope (jag is a big scope, containing many things, Isaac is a way smaller scope and contains only Isaac related things)
Finding the jag::Isaac::Generate method
Nothing much to find, honestly. Remember how there must be a function call at the bottom of our jag::Isaac::Init function? That is our jag::Isaac::Generate method!
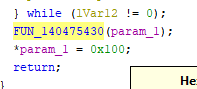
Double-click it to go to the function.
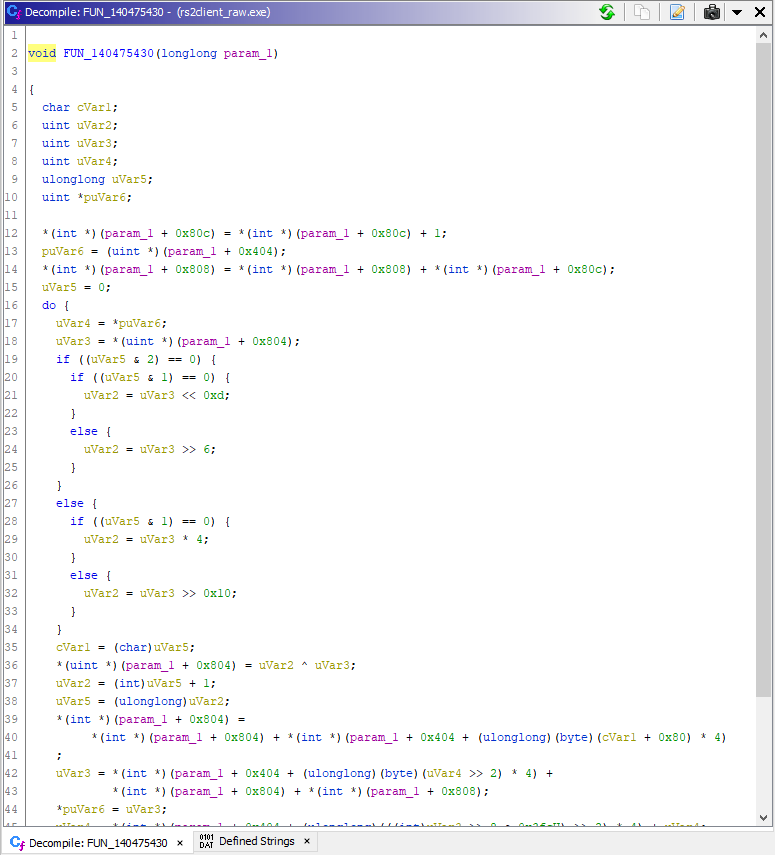
NOTE: If the function does not look like that, please PM me. It could be I made a mistake and that there's two similar functions that do different things. In which case I need to know so I can update this tutorial
First things first, let's rename the function so that we know which function we're dealing with again. Select the function name in the decompiler, press L and enter jag::Isaac::Generate. Now we can get back to this method easily through the Symbol Tree
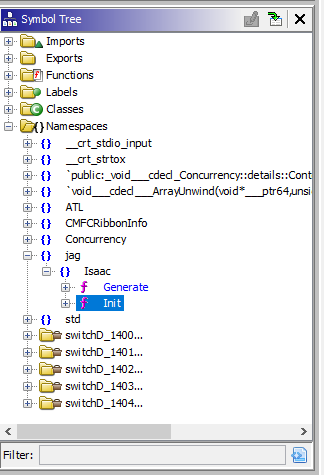
Creating a struct for our jag::Isaac struct
We're decompiling the NXT binary as if it were C, so instead of using classes, we're using structs (To keep things simple). Ghidra does have support to create class structures, but I won't cover that in this tutorial.
In our jag::Isaac::Generate function, scroll to the top of the function and rename param_1 to this. Select param_1 and click L on your keyboard to rename a variable.
Now, this field probably has a random data type, even though we know it's a struct. So let's create a struct for this data type. Right-click either the parameter type or the parameter name and select Auto Create Structure. Ghidra will now automatically create a struct that most of the time has the right size and some of the fields already identified.
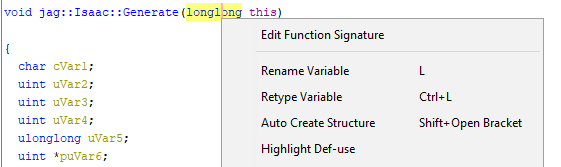
Our struct is now called astruct, but we want it to have an identifiable name. Right-click astruct in the decompiler and click Edit Data Type. This will open a popup that looks something like this:
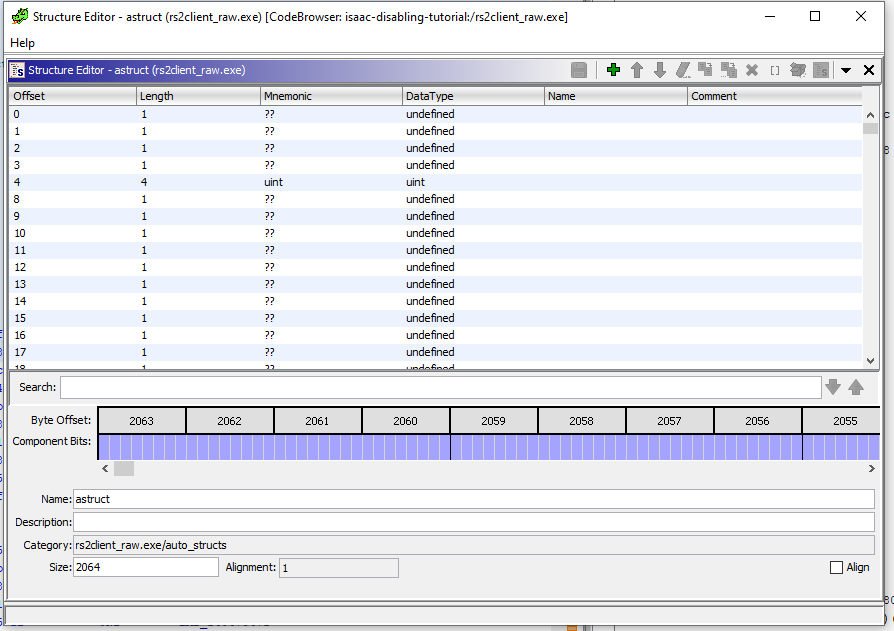
At the bottom there's some fields, we're mainly interested in the Name field. Re-name the struct to jag::Isaac. The size, most of the time, is correct. Now, close the type editor and you'll see our function's prototype is now very readable:

Refactoring our method and struct
Our decompiler window now looks slightly different, with a lot of arrows and fields instead of array indices/pointer magic going on:
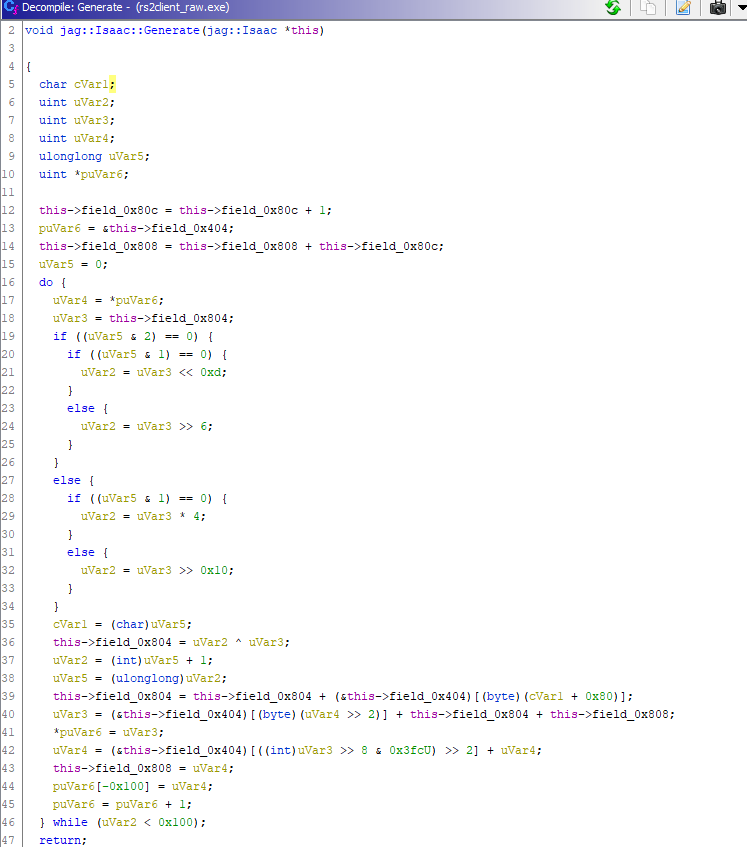
Now comes the fun part - refactoring our Isaac struct. We're now going to be cross-referencing with a normal Isaac implementation:
Code:
private void generateMoreResults() {
cc++;
bb += cc;
for (int i=0; i<256; i++) {
int x = mm[i];
switch (i&3) {
case 0:
aa = aa^(aa<<13);
break;
case 1:
aa = aa^(aa>>>6);
break;
case 2:
aa = aa^(aa<<2);
break;
case 3:
aa = aa^(aa>>>16);
break;
}
aa = mm[i^128] + aa;
int y = mm[i] = mm[(x>>>2) & 0xFF] + aa + bb;
randResult[i] = bb = mm[(y>>>10) & 0xFF] + x;
}
valuesUsed = 0;
}
As you can see, in my decompiler window, line 12 is incrementing something by one, and is used in line 14 to increment another field. So we already know which field is bb and cc. We can rename these fields through the Data Type Editor, or just through the decompiler. Select the variable names, and press L. Now enter the proper names:
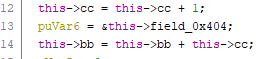
On line 36 we can see a bitwise XOR operation, which looks a lot like the aa = aa ^ (aa *something*) going on in the switch statement above, so we can rename that field to aa.

Based on that, we can also figure out which field is mm - in my code that's field_0x404, as on line 39 aa is incremented using mm. So let's rename that to mm.

Now, that looks quite ugly. Let's open our data editor and see what's going on. We know mm is an array that holds 256 ints. Go to the top of the function, right-click jag::Isaac (The parameter type), click Edit Data Type and in Search enter mm, then press enter:
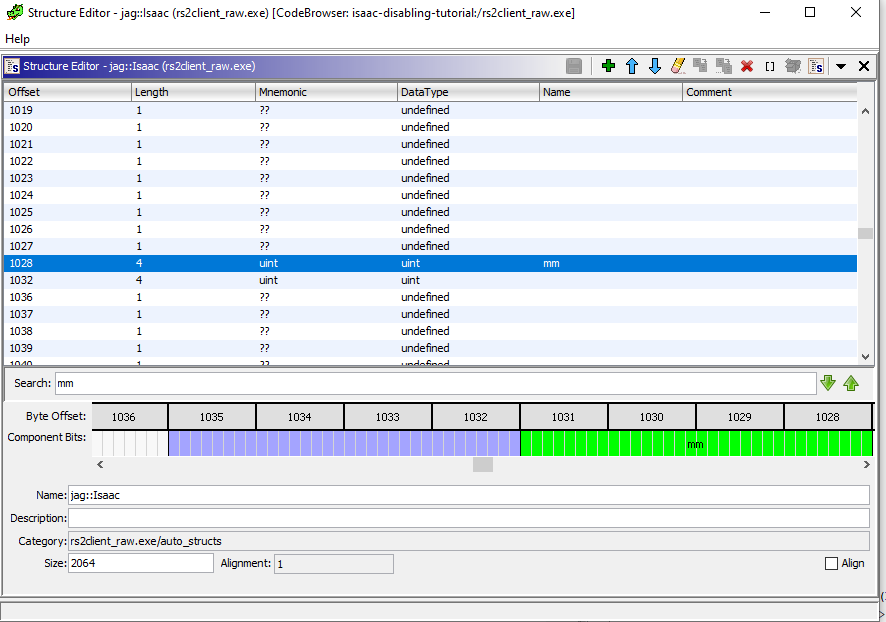
As you can see, Ghidra identified field mm to be an uint, but not a uint array! Let's change it. Let's double-click the DataType column for mm and enter uint[256]:
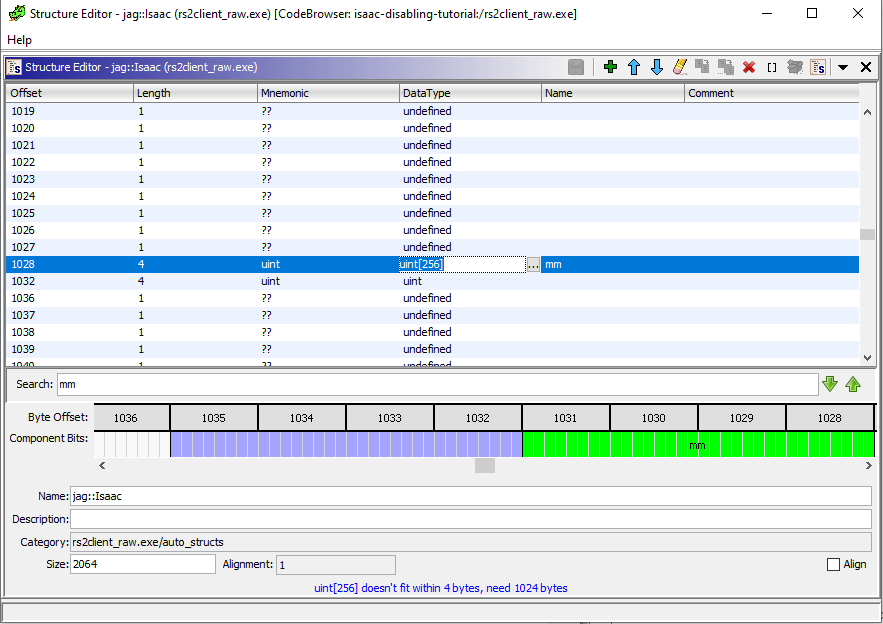
It won't let us press enter. At the bottom, an error pops up uint[256] doesn't fit within 4 bytes, need 1024 bytes. The field below is identified as uint too, causing this error (In my editor at offset 1032). So let's un-identify that field. Press ESC to cancel setting the data type of mm. Now click the field below (For me, offset 1032). Then press C on your keyboard to Clear the field. This sets the bytes to undefined:

Now, let's try to set the type of mm to an uint[256]:

That seems to have done the trick!

Now, there's still some unresolved fields we have to resolve. Let's get on with that! Around line 44 in my decompiler there's this code:

Interestingly enough, the variable puVar6 is set to this->mm at the beginning, which is an array. Also, interestingly enough, hex 0x100 is decimal 256, and since it's an array of uints (size = 4 bytes), it's setting data 1024 bytes before puVar6. It also increments the memory address of puVar6 each time by 4 bytes (Once again, +1 = 4 bytes as the data type of the array is 4 bytes). Based on this we can conclude there's another array of 256 values right before this array. The only unidentified array that remains is the results array. So let's open our data editor again and scroll up all the way:
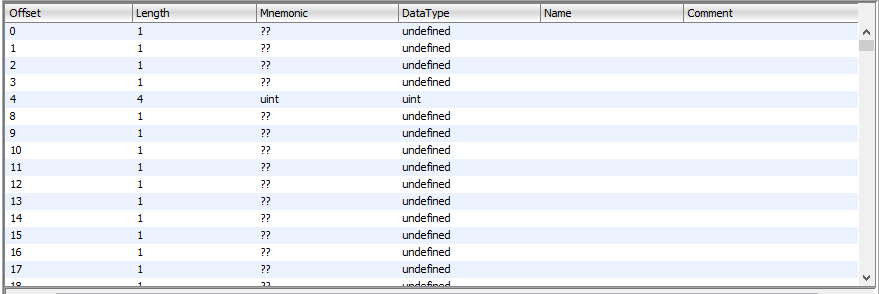
And as you can see, there's already an uint that Ghidra found. Now, let's change the type of this field to an uint[256] just like we did to mm. I have also renamed the field to results by double-clicking the name column:
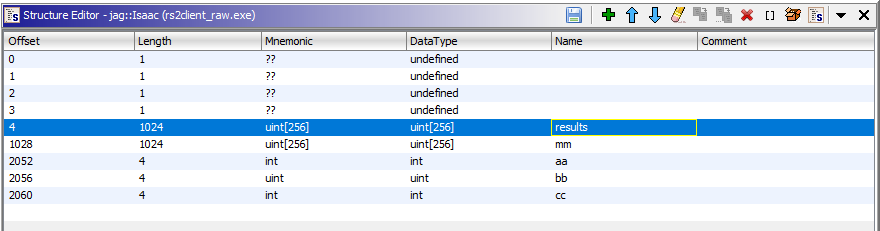
We're almost done completely refactoring the jag::Isaac struct. Only one field remains, which is our remaining field, indicating how many numbers remain before new data must be generated. There's also only enough for one more int, so let's give the field at offset 0 data type uint and let's name it remaining. Now our struct is fully refactored.
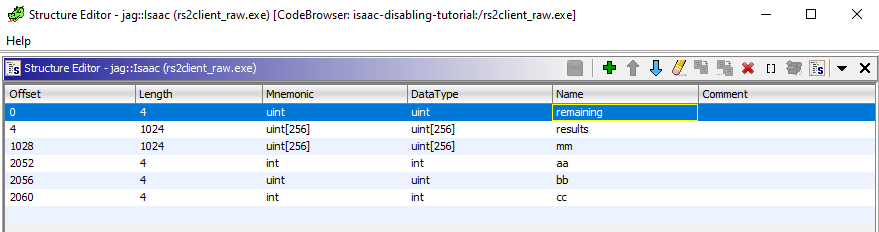
NOTE: Not everything is an int or uint, sometimes fields are char or short. You don't know for sure, but I knew for sure that remaining is of type int. Also the signing on the above fields may be wrong, I just went with what Ghidra picked. The most important thing is having the field names.
Wew. That was definitely a long section, the refactoring part.
Patching ISAAC out
Alright, so now it's time to actually disable ISAAC. For this, we'll be delving into assembly. Remember how the statement here sets a value in the results array?

If we set that to 0, that means every time we get a value for the ISAAC, we get a 0, which means every opcode is offset by 0, effectively removing ISAAC from the protocol, allowing us to be super lazy and making supporting frameworks like Matrix a lot easier.
The question is how we do it, though. Let's click the code in the decompiler, and it'll jump to the related assembly code.
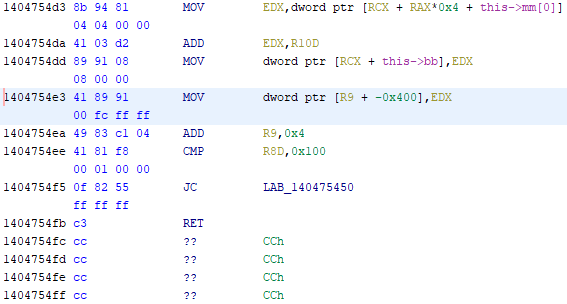
The instruction we're interested in is MOV dword ptr [R9 + -0x400], EDX. This sets the value of dword ptr [R9 + -0x400] to the value of EDX. EDX and R9 are registers (You can google them - assembly x64 registers). Sadly, if we patch this to MOV dword ptr [R9 + -0x400], 0x0, we'll use too many bytes, so that approach is impossible.
We can try to work out if we can change the value of EDX though. It gets assigned and then added to in the first two instructions of the above screenshot. So while we can't do puVar6[-0x100] = 0x0 directly, we can cheat our way there. Instead of doing ADD EDX,R10D we can do XOR EDX,EDX. This is a very memory-efficient way of setting a field to 0, requiring only 2 bytes!
Right-click the ADD EDX,R10D code and click Patch Instruction. You will get a popup about assembler rating, just click OK. It will then construct the assembler. After a bit we can type:

Since we want to set the value in the register EDX to 0, we do XOR in the operator, and as operands, we enter EDX,EDX
Note: Operator = the field on the left. Operand = the field on the right

Now press enter to patch the instruction. But now we have a problem. The previous MOV instruction required 3 bytes, but the XOR only requires 2 bytes. So we have a leftover byte! Thankfully, we can just patch it to NOP, meaning it'll just get skipped.

Right-click the stray byte, Path Instruction and enter NOP in the operator. Leave the operand field empty. Finally, press enter to patch the instruction.

Finally, as you can see in the decompiler window, we see some changes:
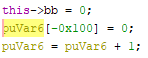
The results array is now completely set to zeroes! It doesn't really matter that we broke the mm field - we're breaking ISAAC anyway. Now, everything is set to zero and packet opcodes will be offset by 0 all the time, both for incoming and outgoing packets.
Note: You'll still have to decrypt the XTEA block during login which follows the RSA block, it contains the username. We only patched out the ISAAC stuff, not the XTEA stuff during login ;)
Exporting the binary
First, save the project. Press CTRL+S or the save button in the top-left of the screen. Then click File (Top left of screen)->Export Program Or press O on your keyboard.
Now, set the format to Binary. Then, select an output file. Ghidra will automatically append .bin to the filename, but you can rename it to .exe. Then, click OK and you're good to go!
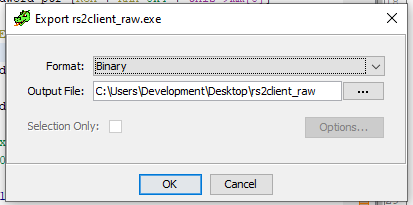
You can now re-calculate your CRC and re-generate your hash, compress the client, and test it out. If you crash due to some stack error, you likely messed something small up. Cry it out and start over. I've done that plenty times.
Finally
I am interested in making more tutorials on NXT, so if you have any ideas, please drop them below. Also, I've only tested this tutorial on the 910 client, so things may look slightly different in other clients. If you're really stuck on another version, please let me know and send me your binary. I can take a look for you. I'm interested in helping the scene out as a whole, and it seems like frameworks have a hard time supporting ISAAC, so this is my first push in the back for those servers. ISAAC can cause a lot of weird issues, especially with the NXT client.
This tutorial also attempts to cover some of the useful parts of reverse engineering, so even if you don't want to patch ISAAC out, it contains a lot of background information that will be required in future tutorials.
↧
↧
Client background
Hello can anyone help me with with Photoshop, just change a Picture to 255 colors since i dont have Photoshop, thanks.
Pm me on discord or here, Tyson#3904
Pm me on discord or here, Tyson#3904
↧
OSRS MQO -> .Dat without use of Datmaker
How can i convert MQO to .dat without datmaker as it corrupts the file!
↧
[Rel] Skeletal Cannon ( Textured & Non-Textured )
Hey all,
Please Help ya self to this model and do what you want with it, Feed back is welcome =)
*Package*
1 x Drop Model
1 x Texture Wear Model
1 x Non-Texture Wear Model
![]()
--- Download Link ----
Please Help ya self to this model and do what you want with it, Feed back is welcome =)
*Package*
1 x Drop Model
1 x Texture Wear Model
1 x Non-Texture Wear Model

--- Download Link ----
↧
↧
667 - make it announce what item player receives?
hi ive added a crystal key on an item chest and i want it to send a game message saying what item the player received :p with the code ive put it announces the item ID not the actual name :/ - code
Spoiler for code:
↧
317 Client stuck reading "client name" is being updated try again in 60 seconds
Hello! My name is Ro, and I'm a total scrub, I have been very interested in making a private server and learning some code therein. That being said, I am working with the Elvarg/OSRSPK client from Prof. Oak.
I was able to download a 188 cache and map, and to my surprise get it to load, *have only seen rev caves/but there are walls now, and I don't clip through them*. Now while in game I entered the command ::update 10 "because it was what the gentleman on YouTube did, and I'm not sure why I did it to begin with." Now the client is stuck saying it's being updated.
I don't want anyone to think that I'm trying to get people to fix things for me, or do anything for me, however I cant seem to find anything related to this specific instance of the server being stuck "updating". I have tried googling and combing the forums here with a few different searches and taglines, to no avail. I would so appreciative if someone could link me a thread where this problem is explained/fixed, or explain to me what I've done. If not hopefully ill figure it out XD.
the command line reads this just before the client starts loading "com.elvarg.game.model.commands.impl.UpdateServer$ 1 execute
INFO: Update task finished!"
Then when I attempt the log in it says "RSPS is being updated. Try again in 60 seconds..."
Thanks for any and help - Ro.
EDIT: UPDATE: I restarted the whole thing, that being eclipse and my computer and it did the trick, but I'm sure my troubles are far from over as this is the beginning of my adventure in code haha. Sorry if I seem dumb, I promise I'm trying.
I was able to download a 188 cache and map, and to my surprise get it to load, *have only seen rev caves/but there are walls now, and I don't clip through them*. Now while in game I entered the command ::update 10 "because it was what the gentleman on YouTube did, and I'm not sure why I did it to begin with." Now the client is stuck saying it's being updated.
I don't want anyone to think that I'm trying to get people to fix things for me, or do anything for me, however I cant seem to find anything related to this specific instance of the server being stuck "updating". I have tried googling and combing the forums here with a few different searches and taglines, to no avail. I would so appreciative if someone could link me a thread where this problem is explained/fixed, or explain to me what I've done. If not hopefully ill figure it out XD.
the command line reads this just before the client starts loading "com.elvarg.game.model.commands.impl.UpdateServer$ 1 execute
INFO: Update task finished!"
Then when I attempt the log in it says "RSPS is being updated. Try again in 60 seconds..."
Thanks for any and help - Ro.
EDIT: UPDATE: I restarted the whole thing, that being eclipse and my computer and it did the trick, but I'm sure my troubles are far from over as this is the beginning of my adventure in code haha. Sorry if I seem dumb, I promise I'm trying.
↧
317 Client crashes when importing custom map (624/625 zip files) for Edgeville edit
So I tried this, i got the maps to show up ingame and all however when I ran inside 1 of the buildings on the map I'm using, it crashed my client and said:
![]()
This is where it crashed ingame:
(This is a "pvp map" of edgeville, downloaded here:
https://www.rune-server.ee/runescape...ville-map.html
![]()
Edit:
I can enter the left of 2 buildings (originally the general store) just fine. The one I am having trouble entering is the one that was the house full of men north of the bank.
Any help would be very highly appreciated, as I'm not finding a lot of information on adding custom maps/map edits.
I followed this video tutorial on how to add the maps.

This is where it crashed ingame:
(This is a "pvp map" of edgeville, downloaded here:
https://www.rune-server.ee/runescape...ville-map.html

Edit:
I can enter the left of 2 buildings (originally the general store) just fine. The one I am having trouble entering is the one that was the house full of men north of the bank.
Any help would be very highly appreciated, as I'm not finding a lot of information on adding custom maps/map edits.
I followed this video tutorial on how to add the maps.
↧
Buying thread layout RSPS
Looking too buy a thread layout for a RSPS, serious offers only, DM me on discord "Codar#1113"
↧
↧
Looking for serious developer for very interesting project (osrs/deob)
Hi everyone,
I am looking for a second SERIOUS developer for our project. Lets start with some details about the project:
- Server base: Started from clean Elvarg, only kept combat skills and reworked almost all other skills to be in line with OSRS. We got a few (+/- 4) skills to go..
- Current client: Weve got a deob client running with one of the more recent Runelite version. All plugins working but all interfaces have to be re-coded. Well most of it is there but we need to convert most of it to use varbits and cs2 scripts.
- Content: We have data up to 184 right now, I have added cerberus as in OSRS including lava pools, ghosts etc. Started on zulrah but might have to rework this.
Maybe some information about me:
I am a 24 years old software engineer from Belgium, graduated in June. I am working about +/- 10 years on RSPSs BUT I would shrink that down to the last 2 years since from that point I really started writing my own systems. Before that I mainly tried to fix some small bugs, repacking some stuff etc. My most important experiences:
- Created full equipment preset system which was loading almost instantly (including prayers and spellbooks).
- Added full cerberus with lava pools, ghosts and full combat script
- Wrote +/- 15 skills from scratch to be in line with OSRS skills
- Added some small minigames such as Rise of The Six
- A bunch of not worth to mentioning bugs or small content fixes
I am very passionated about RSPSs and my dream would be to get this project rolling. Looking for some minimal content finished to be able to release and start pushing out updates every month and fixes every week.
Future vision:�- Content:
- Adding all OSRS bosses such as all wildy bosses etc.
- Adding some minigames
- Adding inferno
- Adding raids (later on)
- Finishing up all interfaces
- Move over equipment presets I made from previous base
- Make bank place holders work
- Etc..
Requirements for becoming our dev:
- Some serious RSPS experience
- Experience with using deob clients
- Experience with varbits/cs2 scripts (should have if you have experience with deob clients)
- Enough free time to put into the project
We have a VERY clean base to start of where there is a decent foundation already for a lot of content. I am looking into this project to make this a beauty but I on my own can simply not handle it. For example, we have all varbits, cs2 scripts, interfaces etc in place, we just need to connect the button clicks to the server. Client is listening for everything already.
Application process:
- Add me on discord: Mr Bobo#8525
- I will have a basic conversation with you
- If I think you might be a good match I will set up a call with you to talk more in detail about your experiences and previous work.
Hope to find YOU soon. We need you 😉
Will prettify this post when I’m at home. At work rn so...
I am looking for a second SERIOUS developer for our project. Lets start with some details about the project:
- Server base: Started from clean Elvarg, only kept combat skills and reworked almost all other skills to be in line with OSRS. We got a few (+/- 4) skills to go..
- Current client: Weve got a deob client running with one of the more recent Runelite version. All plugins working but all interfaces have to be re-coded. Well most of it is there but we need to convert most of it to use varbits and cs2 scripts.
- Content: We have data up to 184 right now, I have added cerberus as in OSRS including lava pools, ghosts etc. Started on zulrah but might have to rework this.
Maybe some information about me:
I am a 24 years old software engineer from Belgium, graduated in June. I am working about +/- 10 years on RSPSs BUT I would shrink that down to the last 2 years since from that point I really started writing my own systems. Before that I mainly tried to fix some small bugs, repacking some stuff etc. My most important experiences:
- Created full equipment preset system which was loading almost instantly (including prayers and spellbooks).
- Added full cerberus with lava pools, ghosts and full combat script
- Wrote +/- 15 skills from scratch to be in line with OSRS skills
- Added some small minigames such as Rise of The Six
- A bunch of not worth to mentioning bugs or small content fixes
I am very passionated about RSPSs and my dream would be to get this project rolling. Looking for some minimal content finished to be able to release and start pushing out updates every month and fixes every week.
Future vision:�- Content:
- Adding all OSRS bosses such as all wildy bosses etc.
- Adding some minigames
- Adding inferno
- Adding raids (later on)
- Finishing up all interfaces
- Move over equipment presets I made from previous base
- Make bank place holders work
- Etc..
Requirements for becoming our dev:
- Some serious RSPS experience
- Experience with using deob clients
- Experience with varbits/cs2 scripts (should have if you have experience with deob clients)
- Enough free time to put into the project
We have a VERY clean base to start of where there is a decent foundation already for a lot of content. I am looking into this project to make this a beauty but I on my own can simply not handle it. For example, we have all varbits, cs2 scripts, interfaces etc in place, we just need to connect the button clicks to the server. Client is listening for everything already.
Application process:
- Add me on discord: Mr Bobo#8525
- I will have a basic conversation with you
- If I think you might be a good match I will set up a call with you to talk more in detail about your experiences and previous work.
Hope to find YOU soon. We need you 😉
Will prettify this post when I’m at home. At work rn so...
↧
How I feel after walking out of the gym
↧
Rank diversification
Code:
Registered Member
↧
BUYING client services
I am looking for these services:
*Login Screen (GFX and Code)
can be purchased by different people
*Custom made launcher with MD5 checksum
*Icon bug fix - Icons do not work in PM, Global.
paying first
Discord: Etheraa#7358
*Login Screen (GFX and Code)
can be purchased by different people
*Custom made launcher with MD5 checksum
*Icon bug fix - Icons do not work in PM, Global.
paying first
Discord: Etheraa#7358
↧
↧
Selling host ready server/client/cache
Hello I'm currently selling my server "Drako" I recently launched it but me being solo on the project has caused me to lose motivation along with me being super busy irl recently I'd like to sell 2 copies for $75 each or I will sell out all the rights to everything for $120(If someone buys everything then I will not sell another copy)
Server features and media can be found here -
https://www.rune-server.ee/runescape...p-ironman.html
The server is still live and can be seen live
Server will come with the discord if purchasing the full server there is currently 52 members in the discord which can be found here
- Discord
Add me one discord to discuss more "F-16#0616"
Server features and media can be found here -
https://www.rune-server.ee/runescape...p-ironman.html
The server is still live and can be seen live
Server will come with the discord if purchasing the full server there is currently 52 members in the discord which can be found here
- Discord
Add me one discord to discuss more "F-16#0616"
↧
317 Death's Server Group Ironman l [CASTLE WARS] [CUSTOM HOME] [content packed]
Website l Forum l Download
![]()
Working
Being Worked on
Not added yet
Bossing
Kbd
God wars
God Wars II [custom]
Alchemical Hydra
Abyssal Sire
Skotizo
Crazy Archeologist
Vetion
Vorkath
Kalphite
Cerberus
Kraken
Callisto
Scorpia
Cerberus
Venenatis
Chaos Fanatic
Zulrah
ancient gorilla
Lizardman Shaman's
Mini Games
Group Ironman
Barrows
Bounty Hunter
Treasure trails
Warriors Guild
Clan Wars
castle wars
Raids
Raids 2
Inferno
Fight caves
Our Team
Zen Aku Owner/Developer
Scyther Owner/Developer
Coins Owner/Developer
Fiery Legion Developer
Tatskaaa Administrator
Zen Aku Owner/Developer
Scyther Owner/Developer
Coins Owner/Developer
Fiery Legion Developer
Tatskaaa Administrator

Working
Being Worked on
Not added yet
Bossing
Kbd
God wars
God Wars II [custom]
Alchemical Hydra
Abyssal Sire
Skotizo
Crazy Archeologist
Vetion
Vorkath
Kalphite
Cerberus
Kraken
Callisto
Scorpia
Cerberus
Venenatis
Chaos Fanatic
Zulrah
ancient gorilla
Lizardman Shaman's
Mini Games
Group Ironman
Barrows
Bounty Hunter
Treasure trails
Warriors Guild
Clan Wars
castle wars
Raids
Raids 2
Inferno
Fight caves
Bank

Shop1

Slayer

Ironman

Thieving

Group Ironman
Shop of a team

Resource area for group ironman

Revs

Raids

Raids wait lobby

Smithing guild

Images from members of our community.
Donator bank note.

Spoiler for To Come:
Spoiler for Previous Discord Update Logs:
↧
718 Models help
Hey, i use blender for modeling
and idk how to upload it to 718 source
i already tried something catchy and some stuff but don't work
it gives me invisibly NPC
Bump
and idk how to upload it to 718 source
i already tried something catchy and some stuff but don't work
it gives me invisibly NPC
Bump
↧
[Averon] new custom home made
well, this is mostly my new home for a project am working on. took me a while to get it but i really like it the way the (city) is comming together. let me know what you guys think about it and perhaps mebe things that should change. much appreciated for taking your time to watch this :D
![]()
![]()
![]()
![]()
![]()
![]()
![]()
![]()
Credits RSPSI
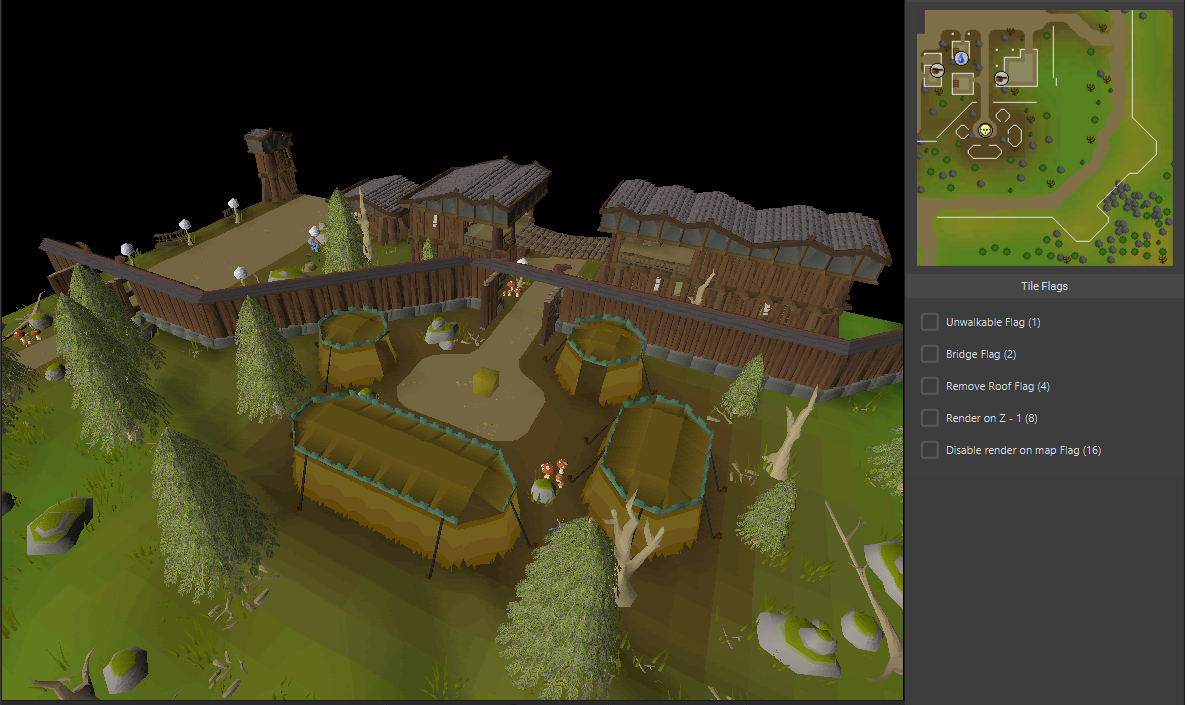
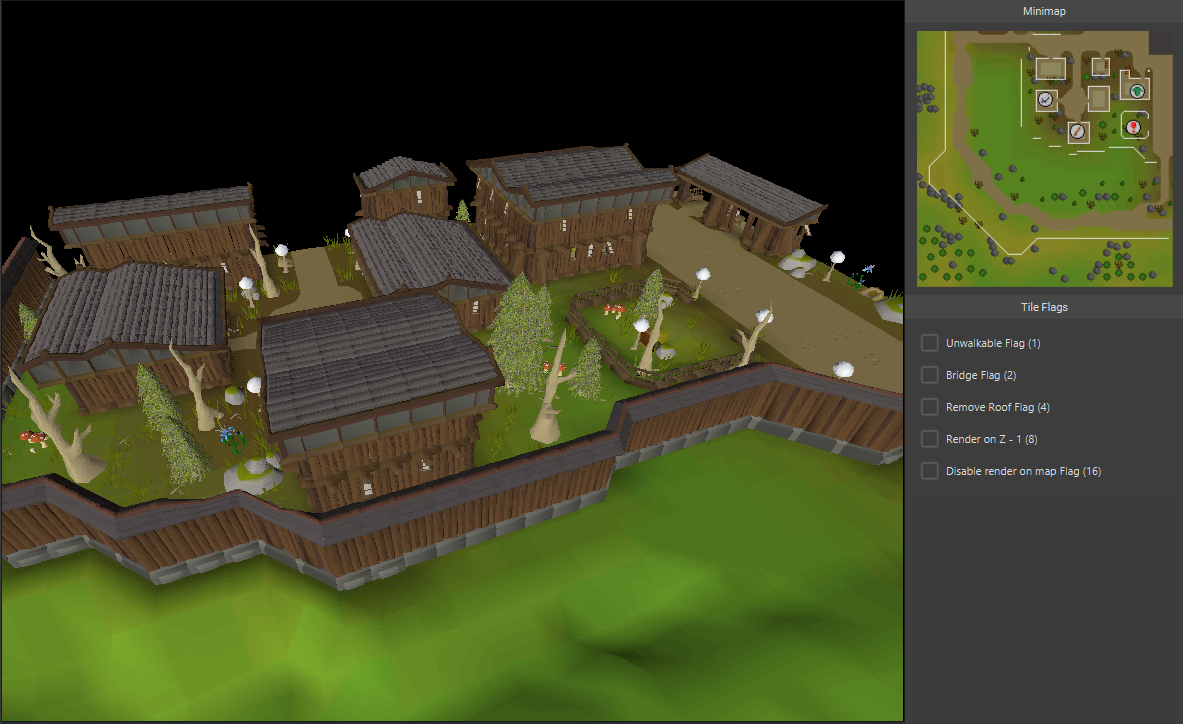
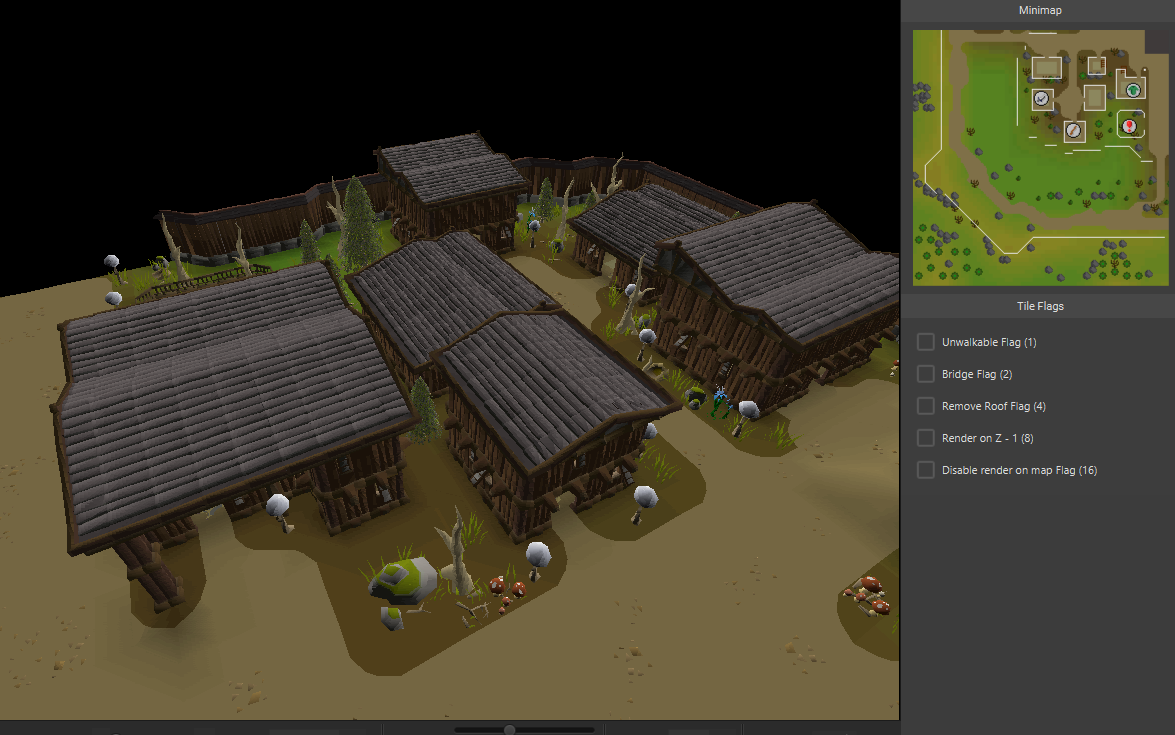
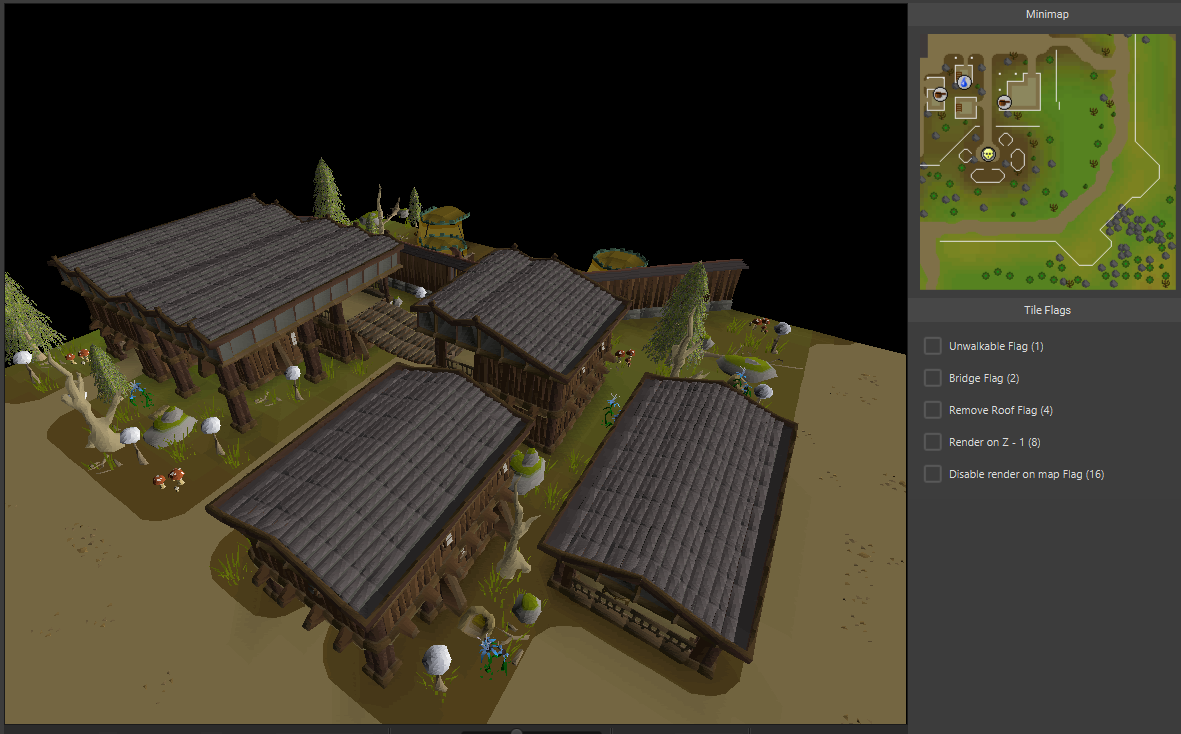
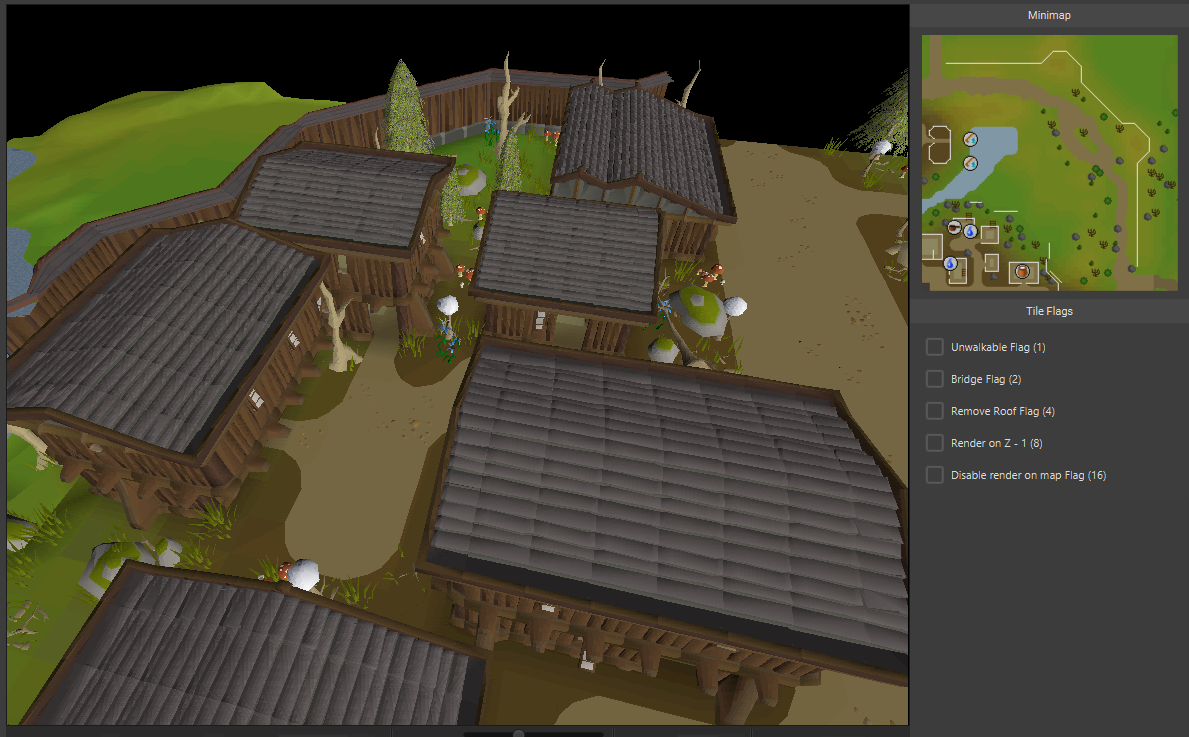
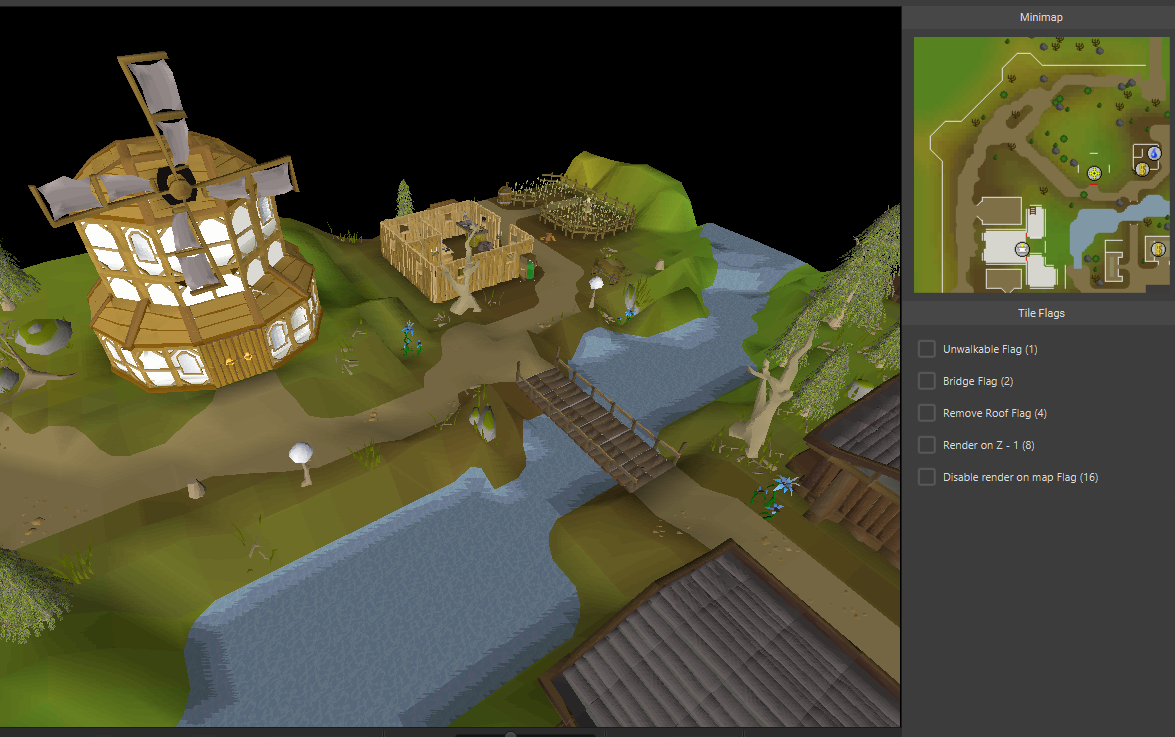
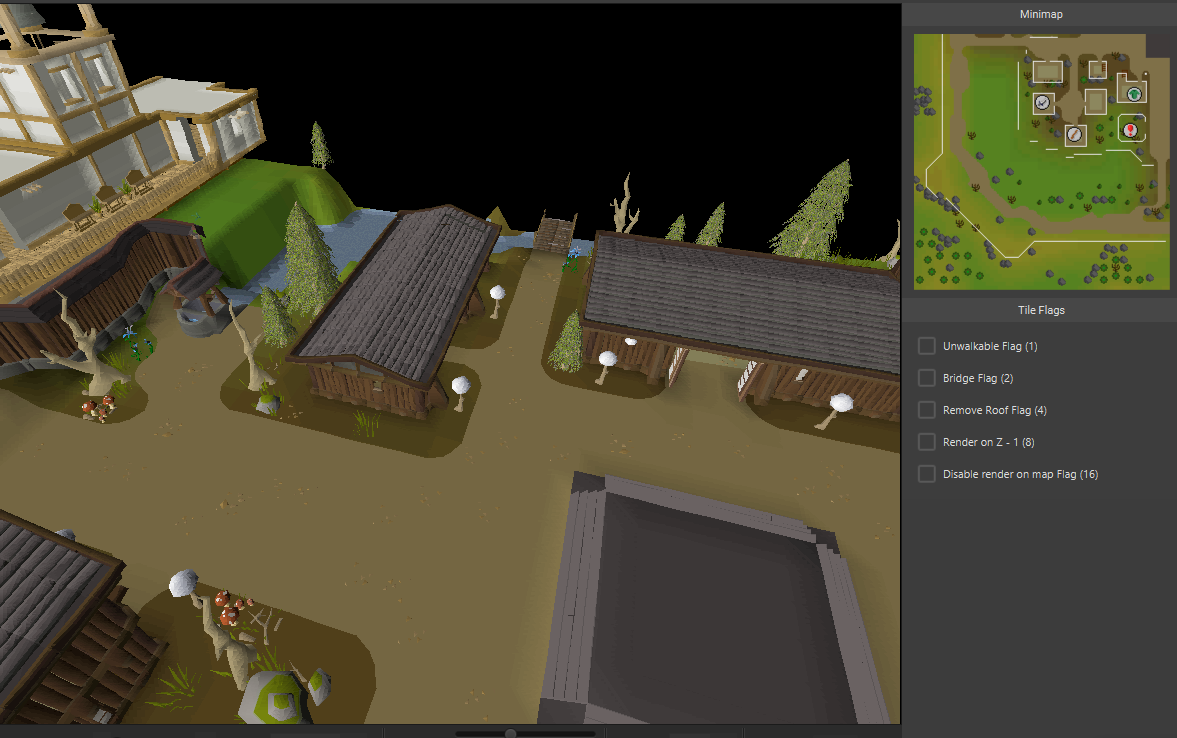
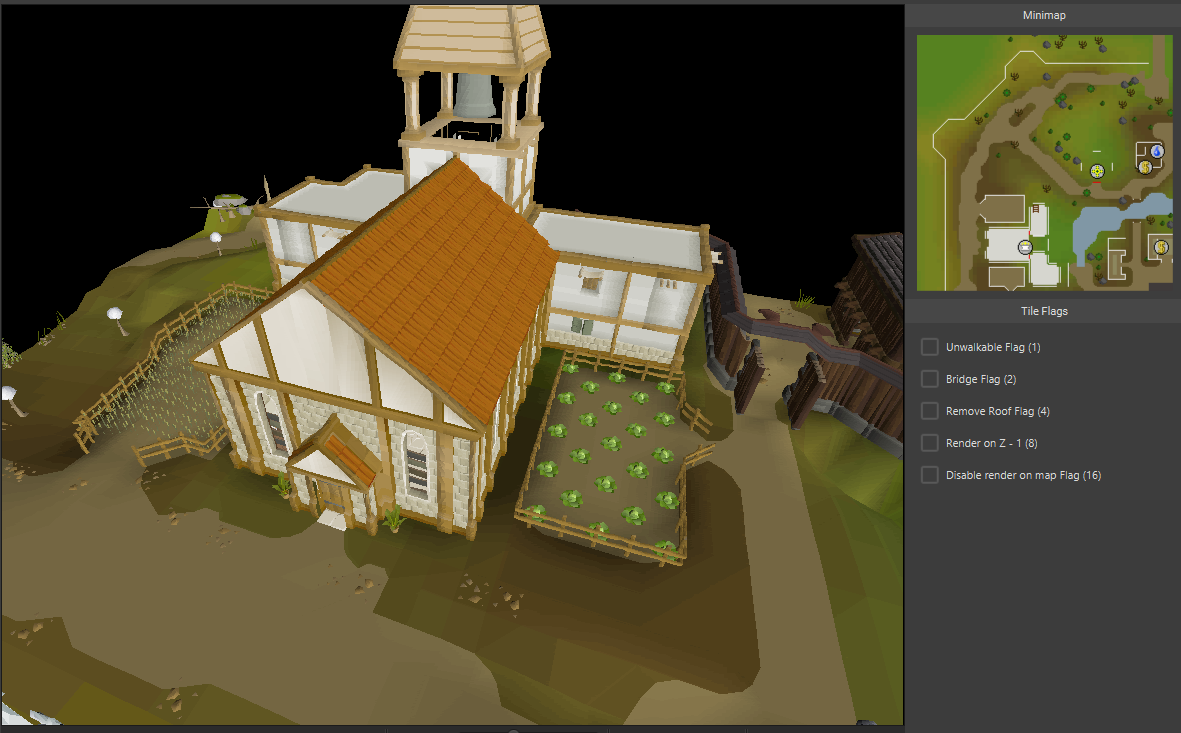
Credits RSPSI
↧
↧
718 New items on 718/901
Guys i tryed to put the 910 items on my 718/901 cache, so far, so good, but when i try to load my client with the cache, my client not load 100% only who i get is 94% loading screen i can't join on the lobby.
Error catched:
Error: ns.f() | Class329.method3993:236 Class255.run:102 java.lang.Thread.run | java.lang.IllegalArgumentException
Error catched:
Error: ns.f() | Class329.method3993:236 Class255.run:102 java.lang.Thread.run | java.lang.IllegalArgumentException
Code:
public boolean method3993(int i, byte[] is, int i_38_, int i_39_) {
try {
synchronized (aClass484_3490) {
if (i_38_ < 0 || i_38_ > -1289609275 * anInt3494)
throw new IllegalArgumentException();
boolean bool = method3991(i, is, i_38_, true, 1494361890);
if (!bool)
bool = method3991(i, is, i_38_, false, 1552417971);
boolean bool_40_ = bool;
return bool_40_;
}
}
catch (RuntimeException runtimeexception) {
throw Class346.method4175(runtimeexception, new StringBuilder().append("ns.f(").append(')').toString());
}
}
↧
317 searching for a funny server to play
Title says it.. Searching for a funny server to play that its Super easy to train and get stuff with good drop rates So recommend me some servers to spend my time in ( NO CUSTOMS )
↧
718 .Obj to .Dat
hey, i need help
i'm using source 718 and Blender for modeling
how can i convert .Obj to .dat i have Datmaker for 317 :/
please help
i'm using source 718 and Blender for modeling
how can i convert .Obj to .dat i have Datmaker for 317 :/
please help
↧